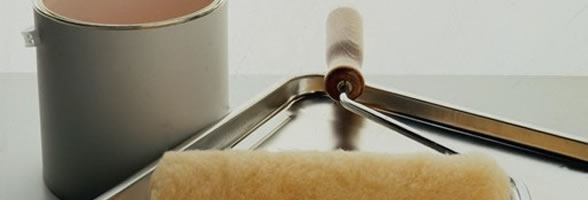
Microbit Python 課程介紹 --Storage
2017-07-22 20:16Microbit Python 課程介紹 --Storage
有時你需要存儲有用的信息。 這樣的信息被存儲為數據:信息的表示(在數字形式存儲在計算機上時,in a digital form when stored on computers)。 如果您將數據存儲在計算機上,那麼即使您關閉設備並重新打開,它仍然會持續存在。
令人高興的MicroPython Micro:Bit允許您使用非常簡單的文件系統執行此操作。 由於內存限制,文件系統上有大約30k的可用存儲空間(there is approximately 30k of storage available )。
什麼是文件系統?
這是一種以持久的方式存儲和組織數據的方式 - 存儲在文件系統中的任何數據都應該能夠在設備的重新啟動後生效。 顧名思義,存儲在文件系統上的數據被組織成文件。
計算機文件是存儲在文件系統上的命名數字資源。 這些資源包含有用的信息作為數據。 這正是紙張文件的工作原理。 它是一種包含有用信息的命名容器。
Usually, both paper and digital files are named to indicate what they contain. On computers it is common to end a file with a .something suffix. Usually, the “something” indicates what type of data is used to represent the information. For example, .txt indicates a text file, .jpg a JPEG image and .mp3 sound data encoded as MP3.
通常,紙和數字文件都被命名為表示它們包含什麼。 在計算機上,通常會以.something suffix 結束文件。 通常,“東西”表示什麼類型的數據用於表示信息。 例如,.txt 表示一個文本文件.jpg一個JPEG圖像和. .mp3聲音數據編碼為MP3。
某些文件系統(例如筆記本電腦或PC上的文件系統)允許您將文件組織到目錄中:將相關文件和子目錄組合在一起的命名容器。 但是,MicroPython提供的文件系統是一個平面文件系統(the file system provided by MicroPython is a flat file system)。 平面文件系統沒有目錄 - 您的所有文件都只存儲在同一個地方。
Python編程語言包含易於使用和強大的方法來處理計算機的文件系統。 micro:bit上的MicroPython實現了這些功能的一個有用的子集,使得易於在設備上讀取和寫入文件,同時也提供與其他版本的Python的一致性。
Warning
Flashing your micro:bit will DESTROY ALL YOUR DATA since it re-writes all the flash memory used by the device and the file system is stored in the flash memory.
However, if you switch off your device the data will remain intact until you either delete it or re-flash the device.
閃爍您的micro:bit將破壞您的所有數據,因為它重新寫入設備使用的所有閃存,並且文件系統存儲在閃存中。
但是,如果關閉設備,數據將保持不變,直到您刪除它或重新閃爍設備。
Open Sesame
在文件系統上讀取和寫入文件是通過打開的功能(the open function)實現的。 一旦打開文件,您可以使用它完成任務,直到你關閉它(類似於我們使用紙張文件的方式)。 關閉一個文件是必不可少的,所以MicroPython知道你已經完成了。
確保這一點的最好方法是使用with語句,如下所示:
with open('story.txt') as my_file:
content = my_file.read()
print(content)
The with statement uses the open function to open a file and assign it to an object. In the example above, the open function opens the file called story.txt (obviously a text file containing a story of some sort). The object that’s used to represent the file in the Python code is called my_file. Subsequently, in the code block indented underneath the with statement, the my_file object is used to read() the content of the file and assign it to the content object.
with語句使用open函數打開一個文件並將其分配給一個對象。 在上面的示例中,打開的函數打開名為story.txt的文件(顯然是一個包含某種故事的文本文件)。 用於表示Python代碼中的文件的對象稱為my_file。 隨後,在with語句下面的代碼塊中,my_file對像用於讀取()文件的內容並將其分配給內容對象。
這裡是重點,下一行包含print語句不縮進(the next line containing the print statement is not indented.)。 與with語句(the with statement)關聯的代碼塊只是讀取文件的單行。 一旦與with語句(the with statement)關聯的代碼塊關閉,那麼Python(和MicroPython)將自動關閉該文件。 這被稱為上下文處理,並且打開的函數(the open function)創建作為文件的上下文處理程序的對象。
Put simply, the scope of your interaction with a file is defined by the code block associated with the with statement that opens the file.
簡單地說,與文件交互的範圍由與打開文件的with語句(the with statement)關聯的代碼塊定義。
困惑?
不用, 我只是說你的代碼應該是這樣的:
with open('some_file') as some_object:
# Do stuff with some_object in this block of code
# associated with the with statement.
# When the block is finished then MicroPython
# automatically closes the file for you.
就像一個紙質文件一樣,打開數字文件有兩個原因:讀取其內容(如上所示)或寫入文件。 默認模式是讀取文件。 如果你想寫一個文件,你需要以下列方式告訴open函數(the open function):
with open('hello.txt', 'w') as my_file:
my_file.write("Hello, World!")
注意'w'參數(the 'w' argument)用於將my_file物件(the my_file object)設置為寫入模式。 您也可以傳遞'r'參數(an 'r' argument)來將文件對象設置為讀取模式,但由於這是默認值,所以它通常被關閉。
將數據寫入文件是通過(您猜到的)寫入方法(write method)來完成的,該方法將要寫入該文件的字符串作為參數。 在上面的示例中,我將文本“Hello,World!”寫入一個名為“hello.txt”的文件。
簡單!
Note
When you open a file and write (perhaps several times while the file is in an open state) you will be writing OVER the content of the file if it already exists.
If you want to append data to a file you should first read it, store the content somewhere, close it, append your data to the content and then open it to write again with the revised content.
當您打開文件並寫入(可能在文件處於打開狀態時可能需要多次),如果文件已存在,則將寫入OVER文件的內容。
如果要將數據附加到文件中,您應該首先閱讀它,將內容存儲在某處,關閉它,將數據附加到內容中,然後再打開以再次修改內容。
While this is the case in MicroPython, “normal” Python can open files to write in “append” mode. That we can’t do this on the micro:bit is a result of the simple implementation of the file system.
而在MicroPython中就是這種情況,“普通”Python可以打開文件以“追加”模式進行寫入。 我們無法在micro:bit上執行此操作是簡單實現文件系統的結果。
OS SOS
除了閱讀和寫入文件,Python可以操縱它們。 您當然需要知道文件系統上的文件,有時您也需要刪除它們。
在常規計算機上,操作系統(如Windows,OSX或Linux)的角色代表Python來管理。 這樣的功能通過一個名為os的模塊(the osmodule)在Python中可用。 由於MicroPython是操作系統,所以我們決定在osmodule(the osmodule)中保持適當的功能,以保持一致性,所以當您在筆記本電腦或Raspberry Pi等設備上使用“常規”Python時,您將會知道在哪裡找到它們。
本質上,您可以執行與文件系統相關的三個操作:列出文件,刪除文件並請求文件大小。
要列出文件系統上的文件,請使用the listdir function功能。 它返回一個字符串列表,指示文件系統上文件的文件名:
import os
my_files = os.listdir()
要刪除文件,請使用the remove function 它需要一個表示要刪除的文件的文件名作為參數的字符串,如下所示:
import os
os.remove('filename.txt')
最後,有時知道文件讀取之前有多大。 要實現這個使用the size function。 像the remove function一樣,它需要一個表示文件名的字符串,該文件的大小要知道。 它返回一個整數(整數),告訴您文件佔用的字節數:
import os
file_size = os.size('a_big_file.txt')
這是非常好的一個文件系統,但如果我們想把文件放在或者關閉設備怎麼辦?
只需使用microfs實用程序!
File Transfer
你在你用於編程BBC micro的計算機上安裝了Python:位,那麼你可以使用一個名為microfs的特殊實用程序(在命令行中使用它時縮寫為ufs )。 有關安裝和使用microfs的所有功能的完整說明,請參見其文檔( in its documentation)
然而,只需四個簡單的命令就可以完成大部分的事情:
$ ufs ls
story.txt
ls子命令(The ls sub-command)列出文件系統上的文件(以通用的Unix命令ls命名,服務於同一功能的ls)。
$ ufs get story.txt
The get sub-command gets a file from the connected micro:bit and saves it into your current location on your computer (it’s named after the get command that’s part of the common file transfer protocol [FTP] that serves the same function).
The get sub-command從連接的micro:bit獲取文件,並將其保存到計算機上的當前位置(以The get sub-command為命令,該命令是作為相同功能的通用文件傳輸協議[FTP]的一部分)。
$ ufs rm story.txt
The rm sub-command可以從連接的micro:bit上的文件系統中刪除命名(它以命名為通用的Unix命令 rm命名,服務於相同的功能)。
$ ufs put story2.txt
Finally, the put sub-command puts a file from your computer onto the connected device (it’s named after the put command that’s part of FTP that serves the same function).
最後,the put sub-command將您的計算機上的文件放在連接的設備上(以the put sub-command為名,這是FTP服務於同一功能的一部分)。
Mainly main.py
文件系統還有一個有趣的屬性:如果您剛剛將MicroPython運行時閃存到設備上,那麼當它啟動時,它只是等待著要做的事情。 但是,如果將一個名為main.py的特殊文件複製到文件系統上,則重新啟動設備後,MicroPython將運行the main.py file的內容。
此外,如果您將其他Python文件複製到文件系統上,那麼您可以像任何其他Python模塊一樣導入它們( import them)。 例如,如果您有一個包含以下簡單代碼的hello.py文件:
def say_hello(name="World"):
return "Hello, {}!".format(name)
...您可以導入並使用這樣的say_hello 函數:
from microbit import display
from hello import say_hello
display.scroll(say_hello())
當然,它會導致文本“Hello,World!”滾動顯示。 重要的一點是,這樣一個例子是在兩個Python模塊之間分割,並且he import statement用於共享代碼。
Note
If you have flashed a script onto the device in addition to the MicroPython runtime, then MicroPython will ignore main.py and run your embedded script instead.
如果除了MicroPython運行時之外,還將腳本閃存到設備上,那麼MicroPython將忽略main.py並運行您的嵌入式腳本。
To flash just the MicroPython runtime, simply make sure the script you may have written in your editor has zero characters in it. Once flashed you’ll be able to copy over a main.py file.
要僅僅使用MicroPython運行時,只需確保編輯器中編寫的腳本中的零字符就可以了。 一旦閃爍,你將能夠複製一個main.py文件。
Microbit 中文 課程 : Python , Javascript, 物聯網
標籤:
—————